What is add_action in WordPress
Add_action
is a powerful tool in WordPress that lets you run your own code at specific points in the WordPress core.
What is add_action
in WordPress? With add_action
, you can hook your custom functions to existing WordPress events or actions.
For example, you could email users when they delete their accounts or add an estimated reading time to your posts.
This flexibility allows you to easily enhance and modify your site without altering the core WordPress files.
Understanding what is add_action
in WordPress and hooks can open up a world of possibilities for your site.
What is add_action in WordPress?
- Hook system function:
add_action
is a core WordPress function used to hook custom functions into WordPress actions. - Extends functionality: It allows you to add custom code at specific points in the WordPress execution without modifying core files.
- Event trigger: Executes your custom function when a specified action (event) occurs in WordPress, such as loading a post or initializing a plugin.
- Customizable and flexible: This enables developers to create dynamic and customizable features for themes and plugins.
- Key to custom development: Essential for developing custom themes and plugins, making WordPress highly extensible and adaptable.
Whether you’re building a simple blog or a complex ecommerce platform, learning what is add_action
in WordPress and how it functions will help you create a more dynamic and personalized user experience.
Understanding actions in WordPress
It’s essential to learn about actions to understand the add_action
function. In WordPress, actions let you add your own code at specific points during execution. They are critical for creating plugins and themes that interact seamlessly with core WordPress functions.
What is an action hook?
An action hook in WordPress is a spot in the core code where you can attach your custom functions.
Using the add_action
function in WordPress, you connect your custom function to these hooks. When WordPress reaches a specific point in its execution, it runs all the functions attached to that hook.
Action hooks allow you to add functionality without altering the core files. This feature keeps your customizations intact even after a WordPress update.
Popular hooks include wp_head
, wp_footer
, and init
. These let you insert code into the header, footer, or at the start of WordPress initialization, respectively.
Common uses of action hooks
Action hooks are widely used in WordPress to enhance your website.
By combining hooks with the add_action
function, you can choose where and when to execute custom functions. This allows you a high degree of control to extend the functionality of your themes and plugins.
For example, you can add custom scripts to your site using the wp_enqueue_scripts
action or manipulate content with the the_content
hook.
Plugins often use action hooks to execute code at precise points.
An ecommerce plugin might use a hook to send confirmation emails after a purchase. Themes can also benefit by using action hooks to add dynamic elements like banners or carousels without hardcoding them.
Using action hooks correctly lets you build complex features while maintaining clean and maintainable code. This makes it a powerful tool in any WordPress developer’s toolkit.
What is add_action in WordPress?
Learning how to use add_action
in WordPress allows you to run custom code when a specific event or hook occurs. This section will offer an overview of what add_action
in WordPress is: how it works, its syntax, how to hook functions to actions, set priorities, and use arguments effectively.
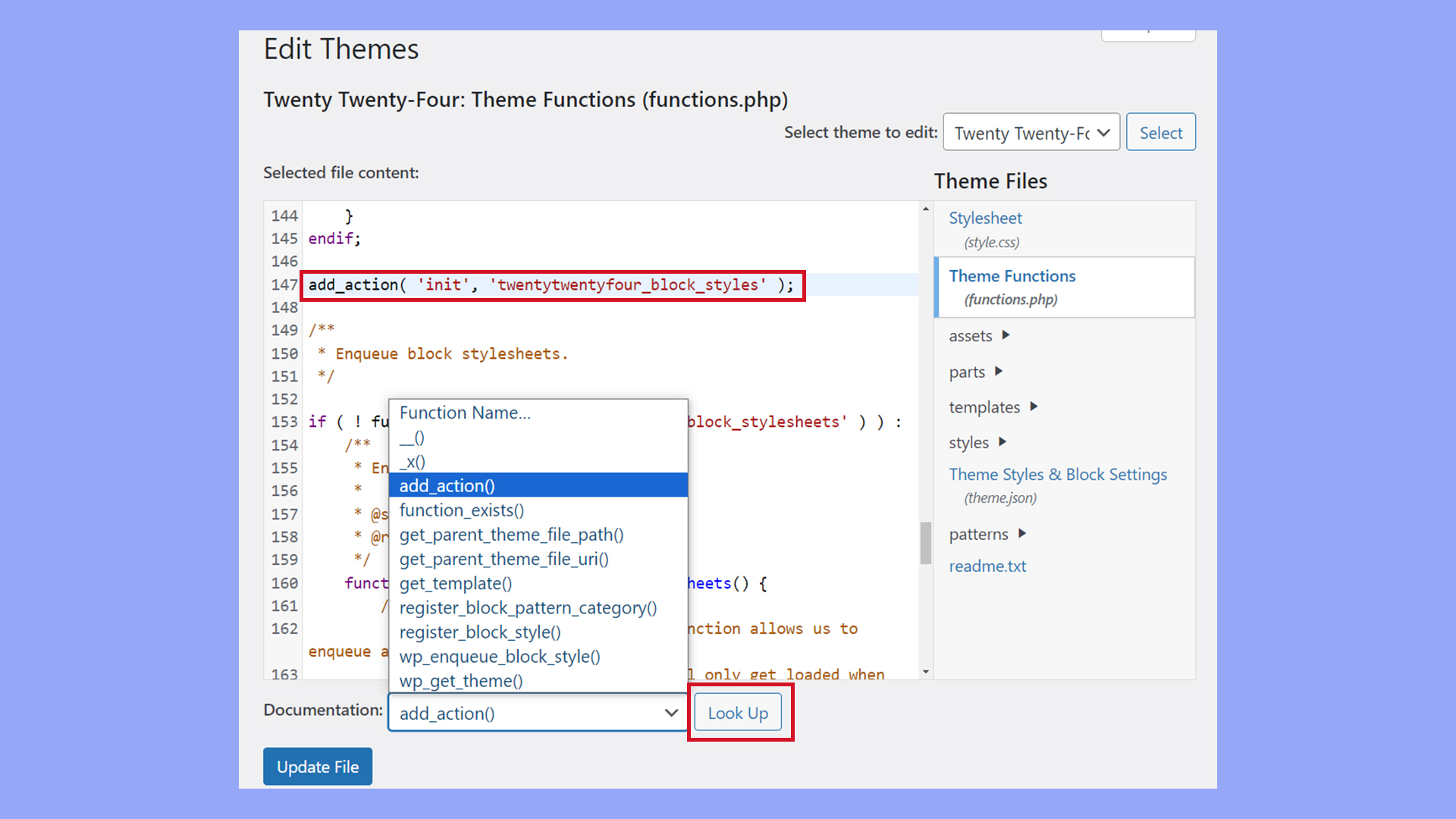
The Theme Editor’s Look Up menu provides quick access to WordPress documentation on some of the most frequently used functions, such as add_action.
Syntax and parameters
The add_action
function sets up your custom function to run at a specific point in WordPress’ execution. It takes several parameters:
add_action($hook_name, $callback, $priority, $accepted_args);
- $hook_name: The name of the hook where you want to attach your function.
- $callback: The name of the function to be executed.
- $priority: Optional. An integer that specifies the order in which the functions associated with a particular hook will be executed. The default is 10.
- $accepted_args: Optional. The number of arguments your function can accept. The default is 1.
Hooking a function to an action
To hook a function to an action, you use add_action
in WordPress.
For example, if you want to display a message in the WordPress header:
function hello_header() { echo "Hello, I’m in the header!"; } add_action('wp_head', 'hello_header');
In this example, hello_header
is the function that triggers when the wp_head
action hook runs. You can replace 'wp_head'
and 'hello_header'
with any hook and function you want.
Prioritizing actions
The priority argument helps you control when your function runs relative to other functions hooked to the same action. Lower numbers run earlier:
add_action('wp_head', 'hello_header', 5); add_action('wp_head', 'another_function', 10);
In this example, hello_header
will run before another_function
because it has a lower priority of 5.
If two functions have the same priority, they will execute in the order they were added.
Using arguments with add_action
Sometimes, the hooked function needs additional arguments. You can specify the number of arguments it can accept with the accepted_args
parameter:
function custom_function($arg1, $arg2) { echo "Argument 1: $arg1"; echo "Argument 2: $arg2"; } add_action('some_hook', 'custom_function', 10, 2);
Working with custom actions
Custom actions in WordPress let you create your own hooks and allow for more flexible development. You can add custom functionalities and ensure your code runs at the right times during WordPress execution.
Creating custom hooks
To create a custom hook, you’ll first need to define it using the do_action
function.
Place this function in your theme’s functions.php
file or in your custom plugin. Here’s an example:
do_action('my_custom_hook');
After defining the custom action, you can attach a callback function to it using add_action
. This callback will execute when the action is fired:
add_action('my_custom_hook', 'my_custom_function'); function my_custom_function() { // Your code here }
Best practices for custom actions
When working with custom actions, it’s important to follow best practices.
First, always prefix your custom hook names to avoid conflicts with other plugins or themes. For instance, use yourprefix_custom_hook
instead of custom_hook
.
Second, keep your callback functions focused and specific. Each function should handle a single task. If needed, break complex tasks into multiple functions.
Third, document your custom hooks and actions. This will help others (and you) understand your code. Comment on each custom action and explain its purpose and usage.
Lastly, ensure you can remove actions if needed using the remove_action
function.
This flexibility allows for cleaner and more maintainable code in the long run. Here’s an example:
remove_action('my_custom_hook', 'my_custom_function');
Extending functionality
WordPress is highly customizable, and the add_action
function plays a crucial role in extending its features.
By using add_action
in WordPress, you can integrate custom functions with themes and plugins, handle form submissions, and add scripts and styles as needed.
Integrating with themes and plugins
When working with WordPress, you often need to connect your custom functions with themes or plugins. Using the add_action
function allows you to hook your code to specific actions taken by WordPress.
For example, if you want to modify the theme’s header, you can use add_action
to tie your function to run when the header loads.
This can be useful for adding custom menus or integrating custom features that need to appear within a theme.
Example:
add_action('wp_head', 'custom_header_content'); function custom_header_content() { echo '<div class="custom-header">Hello World!</div>'; }
Handling forms and submissions
Handling forms and submissions efficiently is critical for many WordPress sites.
With add_action
, you can create custom actions that process form data when a user submits information.
For instance, to handle a contact form submission, you would write a function that processes the form data and then hook it to an action.
This helps ensure your form data is handled properly and avoids potential mishandling of user input.
Example:
add_action('init', 'handle_form_submission'); function handle_form_submission() { if (isset($_POST['submit_form'])) { // Process form data } }
Adding scripts and styles
Adding custom scripts and styles is essential to customizing the look and feel of your WordPress site.
You can efficiently manage your site’s assets by using the add_action
function along with wp_enqueue_script
and wp_enqueue_style
.
These functions allow you to include JavaScript and CSS files in your themes or plugins without hardcoding them into your template files.
This method is preferred as it ensures dependencies are managed properly, and your site remains flexible.
Example:
add_action('wp_enqueue_scripts', 'enqueue_custom_scripts_and_styles'); function enqueue_custom_scripts_and_styles() { wp_enqueue_style('custom-style', get_stylesheet_directory_uri() . '/css/custom-style.css'); wp_enqueue_script('custom-script', get_stylesheet_directory_uri() . '/js/custom-script.js', array('jquery'), '1.0', true); }
In WordPress, using add_action
in these ways helps you extend your site with custom functionality while maintaining a clean and organized codebase.
In conclusion, learning what is add_action
in WordPress and how it works is essential for any developer looking to customize and enhance their website. By using it, you can hook custom functions to specific events, enabling dynamic and flexible site behavior without altering core files.
Whether you’re adding custom scripts, handling form submissions, or creating unique features, using add_action
empowers you to build a more robust and personalized WordPress site. Embrace this powerful tool to streamline your development process and maintain clean, maintainable code.