Defer parsing of JavaScript lets the browser render the web content first and not wait for the JS scripts to finish downloading. It is really an important suggestion that you should not ignore as it might affect the Core Web Vitals (CWV) score of your WordPress site and can reduce your site’s SEO rankings as well. That’s the reason why you should defer the parsing of JavaScript on your WordPress website.
Deferring JavaScript is a key optimization technique used to enhance the performance, speed and SEO rankings of the website. Deferring and parsing are two different things. Parsing means compiling, executing or rendering the JavaScript files by the web browser. Deferring means preventing the script files from executing till the contents of the web page are completely loaded.
Before understanding why we should defer JavaScript, Let’s first understand how JavaScript as a language is interpreted by the web browser. Since browsers work on a single thread which means one single line of code or a statement can be executed at a time, it will start rendering the HTML content line by line and then as soon as it finds some scripts, it executes those scripts first completely and then it resumes executing the remaining HTML content on the screen.
So, If you don’t implement the defer JavaScript technique then while loading the web page content if the browser finds a JavaScript file then it will start downloading that file and render the JavaScript code. This in many cases can affect the performance of the WordPress website as there can be many script files a website needs to load and that’s where the defer JavaScript technique can help.
Why should you defer the parsing of Javascript?
Whenever you use any of the page speed testing tools for testing the speed of your WordPress website you might have come across a suggestion to “defer parsing of JavaScript” on your site.
Defer parsing of JavaScript lets the browser render the web content first and not wait for the JS scripts to finish downloading. It is really an important suggestion that you should not ignore as it might affect the Core Web Vitals (CWV) score of your WordPress site and can reduce your site’s SEO rankings as well.
That’s the reason why you should defer the parsing of JavaScript on your WordPress website. When you defer the loading of JavaScript it affects the below metrics of the Core Web Vitals (CWV).
- Largest Contentful Paint (LCP)
Largest Contentful Paint (LCP) is one of the CWV metrics that determines how much time it takes for a browser to render the largest element on the screen. If the JS is deferred then it can help the browser to focus on rendering the other elements on the screen.
- First Input Delay (FID)
First Input Delay (FID) is one of the CWV metrics that determines how much time it takes for a browser to respond to the first user click on the web page. If the browser is busy executing the JavaScript files then definitely it will take a bit longer time for a browser to respond to user activity. Deferring JavaScript helps improve the FID metric.
Eventually, when all these metrics, LCP, FID and CLS are improved then this also improves the overall Core Web Vitals (CWV) score which results in the following. Apart from this, it also improves other metrics as well, such as TTI.
- Higher SEO rankings
- Faster page load time
- Reduce bounce rate
- Increases conversion rate
- Better user experience
If you want to learn more about CWV metrics then you can check out our article on Core Web Vitals.

Related Articles
What care should be taken?
Make sure that you don’t do the following while deferring parsing of JavaScript.
- Don’t defer JS files which have not-deferred dependent scripts, which depend on them to run properly. The rule of thumb is if script B depends on A, then you cannot defer only script A. But if you do so then the page will not work as expected and it might throw some errors as well.
- Don’t defer JQuery files
- Don’t defer files that are responsible for rendering the above-the-fold content, particularly – the FCP (First Contentful Paint).
When not do defer JS?
If the src attribute is absent on the script tag then the defer attribute must not be used (i.e. for inline scripts), as it would have no effect.
The defer attribute has no effect on module scripts — they defer by default. The module scripts are nothing but a javascript program that is divided into smaller modules and can be imported as and when needed.
Defer JS vs delay JS
You might have come across these two terms defer and delay while reading about defer parsing JavaScript. These terms might sound similar but they are not the same.
Defer JavaScript is a technique where the browser parallelly downloads the scripts while executing HTML content and after it completes executing the HTML content, it starts with parsing and executing of the deferred JavaScript files and updating and re-rendering the DOM elements if these scripts change anything in the DOM.
Delay JavaScript is a technique where we initially only focus on rendering the HTML content and not the script files. To achieve this in practice, on the “script” tag, instead of the “src” attribute we provide it with some other name say “delay” (you can provide any name to this attribute, it does not matter).
Since there is no “src” attribute on the script tag the browser will completely ignore these script tags. Now as soon as the user starts interacting with the webpage we then replace the “delay” with the name “src” so that the browser can now download and execute all these scripts.
const autoLoadDuration = 5; //In Seconds const eventList = ["keydown", "mousemove", "wheel", "touchmove", "touchstart", "touchend"]; const autoLoadTimeout = setTimeout(runScripts, autoLoadDuration * 1000); eventList.forEach(function(event) { window.addEventListener(event, triggerScripts, { passive: true }) }); function triggerScripts() { runScripts(); clearTimeout(autoLoadTimeout); eventList.forEach(function(event) { window.removeEventListener(event, triggerScripts, { passive: true }); }); } function runScripts() { document.querySelectorAll("script[delay]").forEach(function(scriptTag) { scriptTag.setAttribute("src", scriptTag.getAttribute("delay")); }); }
The above code basically takes all the script tags with the “delay” attribute and then replaces them with the “src”. This function runs after a 5 seconds delay after the page is loaded or on user interaction with the page, e.g. mouse moving or scrolling or keyboard press. You can change the delay time based on your requirements.
Which is better or more effective?
The defer JS technique is much more popular as compared to delay JS but both are used in the industry. As we have already seen both these techniques have minor differences. Since in defer JS we parallelly download the JS files while rendering HTML it saves us time as compared to delay JS where after rendering the HTML we then download and execute the JS files.
Async vs Defer attributes
Async and Defer attributes allow the browser to download the JavaScript files simultaneously while the browser is still parsing the HTML content. But there is a slight difference between the two. Let’s now see how both these work and what makes them different.
The Async attribute tells the browser to download the script file parallelly as it parses the HTML content. When the file is completely downloaded it should start executing that script file. After all, the contents of the script file are executed then only continue with the remaining HTML parsing.
You can tell the browser to download a script file asynchronously by adding an “async” keyword within the script tag as shown below.
Whereas, Defer also tells the browser to download the script files parallelly but only execute those files when all the HTML parsing is done. You can add a “defer” keyword on the script tag to tell the browser to defer the script files as follows.
How to defer parsing of Javascript in WordPress?
Functions.php
To defer parsing of JavaScript in WordPress, you can add a few lines of code inside your website’s “functions.php” file from the WordPress admin panel. This approach is for those who don’t want to use external plugins and have some understanding of the WordPress platform.
It’s always recommended to take a backup of your site before making any changes to the “functions.php” file.
function defer_parsing_of_js( $url ) { if ( is_user_logged_in() ) return $url; //don't break WP Admin if ( FALSE === strpos( $url, '.js' ) ) return $url; if ( strpos( $url, 'jquery.js' ) ) return $url; return str_replace( ' src', ' defer src', $url ); } add_filter( 'script_loader_tag', 'defer_parsing_of_js', 10 );
WordPress plugins
If you want to defer the JavaScript files in WordPress but you are not a tech savvy person then no worry, there are lots of WordPress plugins out there to help you.
Varvy method
The other way to defer parsing of JavaScript in WordPress is by adding the Varvy method provided by Patrick Sexton, CEO of Varvy which tells the browser to wait till the page is completely loaded and then both download and execute the JavaScript. Below is the code snippet for varvy method.
function downloadJSAtOnload() { var element = document.createElement("script"); element.src = "defer.js"; document.body.appendChild(element); } if (window.addEventListener) window.addEventListener("load", downloadJSAtOnload, false); else if (window.attachEvent) window.attachEvent("onload", downloadJSAtOnload); else window.onload = downloadJSAtOnload;

Want to speed up your website instantly?
Get 90+ PageSpeed Score automatically with 10Web Booster ⚡
On any hosting!
Speed up your website instantly
Speed up your website instantly
-
Automatically get 90+ PageSpeed score
-
Experience full website caching
-
Pass Core Web Vitals with ease
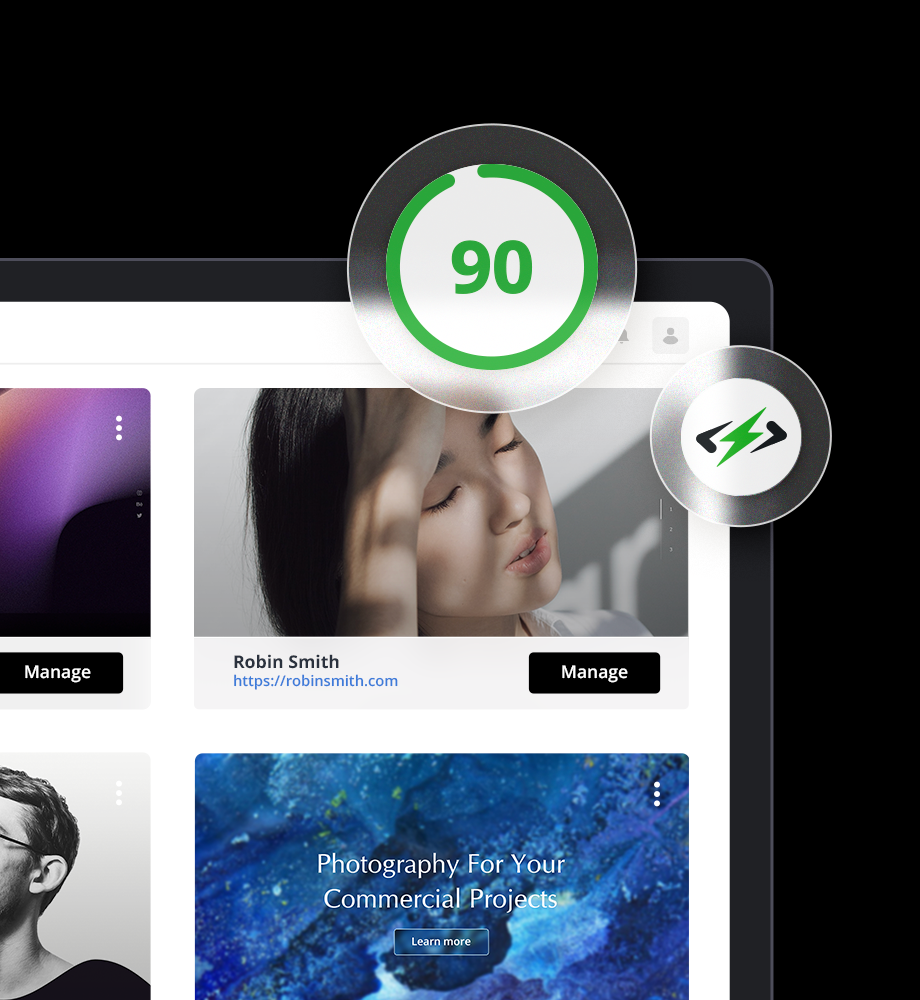